今回はObjective-Cで一覧表示されている各UITableViewCellの中に、さらにUITableViewCellの一覧を表示するやり方について解説します。
かなり大長編となりますが、良ければお付き合いください。
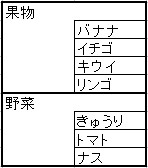
例としては、左図のようなイメージです。
果物→野菜とリストになっていますが、それぞれのセル内に「バナナ→イチゴ→…」とさらに一覧が表示されています。
※当記事は、入れ子になっていない一覧表示はできる技術を前提に進めさせていただきます。
コード例.
「.h/.m/.xib」の3ファイルを2種類準備する
まずは、ストーリーボードでセル用ファイルをそれぞれ2種類作成します。
※今回はわかりやすくするために、セルごとに「.h/.m/.xib」の3ファイルを作成します。
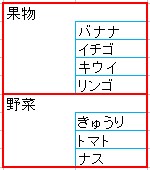
赤枠のUITableViewCellと青枠のUITableViewCellを追加します。
赤枠の「.xib」ファイルには、UITableViewを設置することを忘れないようにしてください。
コードの実装
今回はAPIからJsonObjectを取得したときのコードを記述します。
JsonObjectは、「result_data」から赤枠の一覧を取得でき、赤枠の一覧の中の「detail_info」から青枠のデータが取得できるものとします。
※コードの基本部分は省略しています。
APIからの戻り値のJsonObject
{“Response”:
{“result_data”:
[{“detail_info”:[“バナナ”, “イチゴ”, “キウイ”, “リンゴ”]},
{“detail_info”:[“きゅうり”, “トマト”, “ナス”]}]
}
}
ViewController.h
#import <UIKit/UIKit.h>
@interface ViewController
@property(weak, nonatomic) IBOutlet UITableView *tableView;
@property(nonatomic) NSMutableArray *dataArray;
@end
ViewController.m
-(void)viewDidLoad{
self.tableView.dataSource = self;
self.tableView.delegate = self;
UINib *nib = [UINib nibWithNibName:@”CellName1″ bundle:nil];
[self.tableView registerNib:nib forCellReuseIdentifier:@”CellName1″];
}
-(void)didReceiveResponse:(NSDictionary *)responseDic delegate:(id<DataModelDelegate>)delegate identifier:(NSString *)identifier {
//APIから結果を取得する
dataArray = [NSMutableArray array];
for(NSDictionary *dic in responceDic[@”result_data”]){
NSMutableDictionary *mutableDic = [NSMutableDictionary dictionaryWithDictionary:dic];
[dataArray addObject:mutableDic];
}
}
-(UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath{
UITableViewCell *cell = [self.tableView dequeueReusableCellWithIdentifier:@”CellName1″];
//データから青枠のリストを取得し、セルにセットする。
NSMutableArray *detailList = [NSMutableArray array];
for(NSMutableDictionary *item in dataArray[indexPath.row][@”detail_info”]){
[detailList addObject:item];
}
[cell setDetailData:detailList];
return cell;
}
CellName1.h
#import <UIKit/UIKit.h>
@interface CellName : UITableViewCell
@property(weak, nonatomic) IBOutlet UITableView *tableView;
@property(nonatomic) NSMutableArray *dataArray;
-(void)setDetailData(NSMutableArray *)detailData
@end
CellName1.m
-(void)awakeFromNib{
self.tableView.dataSource = self;
self.tableView.delegate = self;
UINib *nib = [UINib nibWithNibName:@”CellName2″ bundle:nil];
[self.tableView registerNib:nib forCellReuseIdentifier:@”CellName2″];
}
-(UITableViewCell *)tableView:(UITableViewCell *)tableView cellForRowAtIndexPth:(NSIndexPath *)indexPath{
SearchCategoryTreeCell *cell = self.tableView dequeueReusableCellWithIdentifier:@”CellName2″ forIndexPath:indexPath];
[cell setName:dataArray[indexPath.row][@”CellName2″]];
return cell;
}
-(void)setDetailData(NSMutableArray *)detailData{
dataArray = detailData;
[self.tableView reloadData];
}
CellName2.h
@interface CellName2 : UITableViewCell
@property (weak, nonatomic) IBOutlet UILabel label;
-(void)setName:(NSString *)name;
@end
CellName2.m
-(void)setName:(NSString *)name{
[self.label setText:name forState:UIControlStateNormal];
}